Within the age of data overload, it is simple to get misplaced within the wealth of content material accessible on-line. YouTube gives billions of movies and the Web is filled with articles, blogs and tutorial papers. With such a big quantity of information, it’s typically tough to extract helpful data with out spending hours studying and searching. That is the place AI-powered net summarizer comes to assist.
On this article, let’s create a Streamlit based mostly utility utilizing NLP and AI that summarizes YouTube movies and web sites into extremely detailed summaries. This app makes use of Groq Mannequin flame-3.2 and LangChain abstract chains to offer extremely detailed summaries, saving the reader time with out lacking any factors of curiosity.
Studying outcomes
- Perceive the challenges of data overload and the advantages of AI-powered summarization.
- Learn to create a Streamlit app that summarizes content material from YouTube and web sites.
- Discover the position of LangChain and Llama 3.2 in producing detailed content material summaries.
- Learn to combine instruments like yt-dlp and UnstructuredURLLoader for media processing.
- Create a strong net summarizer utilizing Streamlit and LangChain to immediately summarize YouTube movies and web sites.
- Create an internet summarizer with LangChain to get concise and correct content material summaries from URLs and movies.
This text was revealed as a part of the Information Science Blogathon.
Function and Advantages of Summarizer App
From YouTube to net posts to in-depth analysis articles, this huge repository of data is actually at your step. Nonetheless, for many of us, the time issue guidelines out shopping movies that final a number of minutes or studying lengthy articles. In response to research, an individual spends only some seconds on a web site earlier than deciding whether or not to proceed to learn it or not. Now, right here is the issue that wants an answer.
Enter AI-powered summarization: a way that enables AI fashions to digest massive quantities of content material and supply concise, human-readable summaries. This may be particularly helpful for busy professionals, college students, or anybody who needs to rapidly perceive the essence of content material with out spending hours on it.
Abstract Utility Elements
Earlier than we dig into the code, let’s take a look at the important thing components that make this app work:
- LangChain: This highly effective framework simplifies the method of interacting with nice language fashions (LLM). It supplies a standardized solution to handle prompts, chain collectively totally different language mannequin operations, and entry quite a lot of LLMs.
- illuminated: This open supply Python library permits us to rapidly create interactive net functions. It’s simple to make use of and that makes it excellent for creating the interface of our summarizer.
- yt-dlp: When summarizing YouTube movies, yt_dlp is used to extract metadata corresponding to title and outline. In contrast to different YouTube downloaders, yt_dlp is extra versatile and helps a variety of codecs. It’s splendid for extracting video particulars, that are then entered into the LLM for abstract.
- Unstructured URL Loader: This LangChain utility helps us load and course of web site content material. It handles the complexities of looking out net pages and extracting their textual data.
Creating the app: step-by-step information
On this part, we’ll stroll by way of every stage of growing your AI abstract app. We are going to cowl establishing the surroundings, designing the person interface, implementing the abstract mannequin, and testing the applying to make sure optimum efficiency.”
Word: Get the Requisitos.txt file and the total code on GitHub right here.
Importing libraries and loading surroundings variables
This step includes configuring the important libraries required for the applying, together with machine studying and NLP frameworks. We will even load surroundings variables to securely handle API keys, credentials, and configuration settings required all through the event course of.
import os
import validators
import streamlit as st
from langchain.prompts import PromptTemplate
from langchain_groq import ChatGroq
from langchain.chains.summarize import load_summarize_chain
from langchain_community.document_loaders import UnstructuredURLLoader
from yt_dlp import YoutubeDL
from dotenv import load_dotenv
from langchain.schema import Doc
load_dotenv()
groq_api_key = os.getenv("GROQ_API_KEY")
This part imports libraries and masses the API key from an .env file, which retains delicate data corresponding to API keys secure.
Designing the frontend with Streamlit
On this step, we’ll create an interactive and easy-to-use interface for the applying utilizing Streamlit. This contains including enter kinds, buttons, and displaying outcomes, permitting customers to seamlessly work together with backend functionalities.
st.set_page_config(page_title="LangChain Enhanced Summarizer", page_icon="🌟")
st.title("YouTube or Web site Summarizer")
st.write("Welcome! Summarize content material from YouTube movies or web sites in a extra detailed method.")
st.sidebar.title("About This App")
st.sidebar.data(
"This app makes use of LangChain and the Llama 3.2 mannequin from Groq API to offer detailed summaries. "
"Merely enter a URL (YouTube or web site) and get a concise abstract!"
)
st.header("Find out how to Use:")
st.write("1. Enter the URL of a YouTube video or web site you want to summarize.")
st.write("2. Click on **Summarize** to get an in depth abstract.")
st.write("3. Benefit from the outcomes!")
These strains set the web page settings, title, and welcome textual content for the principle person interface of the applying.
Textual content enter for URL and mannequin loading
Right here, we’ll arrange a textual content enter subject the place customers can enter a URL to investigate. Moreover, we’ll combine the required mannequin loading performance to make sure that the applying can course of the URL effectively and apply the machine studying mannequin as wanted for evaluation.
st.subheader("Enter the URL:")
generic_url = st.text_input("URL", label_visibility="collapsed", placeholder="https://instance.com")
Customers can enter the URL (YouTube or web site) they wish to summarize in a textual content entry subject.
llm = ChatGroq(mannequin="llama-3.2-11b-text-preview", groq_api_key=groq_api_key)
prompt_template = """
Present an in depth abstract of the next content material in 300 phrases:
Content material: {textual content}
"""
immediate = PromptTemplate(template=prompt_template, input_variables=("textual content"))
The mannequin makes use of a message template to generate a 300-word abstract of the supplied content material. This template is included into the abstract chain to information the method.
Definition of operate for importing YouTube content material
On this step, we’ll outline a operate that’s chargeable for looking out and loading YouTube content material. This characteristic will take the supplied URL, extract related video knowledge, and put together it for evaluation utilizing the in-app machine studying mannequin.
def load_youtube_content(url):
ydl_opts = {'format': 'bestaudio/greatest', 'quiet': True}
with YoutubeDL(ydl_opts) as ydl:
data = ydl.extract_info(url, obtain=False)
title = data.get("title", "Video")
description = data.get("description", "No description accessible.")
return f"{title}nn{description}"
This operate makes use of yt_dlp to extract data from YouTube video with out downloading it. Returns the title and outline of the video, which might be summarized by the LLM.
Dealing with abstract logic
if st.button("Summarize"):
if not generic_url.strip():
st.error("Please present a URL to proceed.")
elif not validators.url(generic_url):
st.error("Please enter a legitimate URL (YouTube or web site).")
else:
attempt:
with st.spinner("Processing..."):
# Load content material from URL
if "youtube.com" in generic_url:
# Load YouTube content material as a string
text_content = load_youtube_content(generic_url)
docs = (Doc(page_content=text_content))
else:
loader = UnstructuredURLLoader(
urls=(generic_url),
ssl_verify=False,
headers={"Person-Agent": "Mozilla/5.0"}
)
docs = loader.load()
# Summarize utilizing LangChain
chain = load_summarize_chain(llm, chain_type="stuff", immediate=immediate)
output_summary = chain.run(docs)
st.subheader("Detailed Abstract:")
st.success(output_summary)
besides Exception as e:
st.exception(f"Exception occurred: {e}")
- If it is a YouTube hyperlink, load_youtube_content extracts the content material, wraps it in a doc, and shops it in paperwork.
- If it’s a web site, UnstructuredURLLoader retrieves the content material as paperwork.
Operating the abstract string: The LangChain abstract chain processes the uploaded content material and makes use of the request template and LLM to generate a abstract.
To provide your app a classy look and supply important data, we’ll add a customized footer utilizing Streamlit. This footer can show necessary hyperlinks, thank yous or contact particulars, making certain a clear {and professional} person interface.
st.sidebar.header("Options Coming Quickly")
st.sidebar.write("- Choice to obtain summaries")
st.sidebar.write("- Language choice for summaries")
st.sidebar.write("- Abstract size customization")
st.sidebar.write("- Integration with different content material platforms")
st.sidebar.markdown("---")
st.sidebar.write("Developed with ❤️ by Gourav Lohar")
Manufacturing
Enter: https://www.analyticsvidhya.com/weblog/2024/10/nvidia-nim/
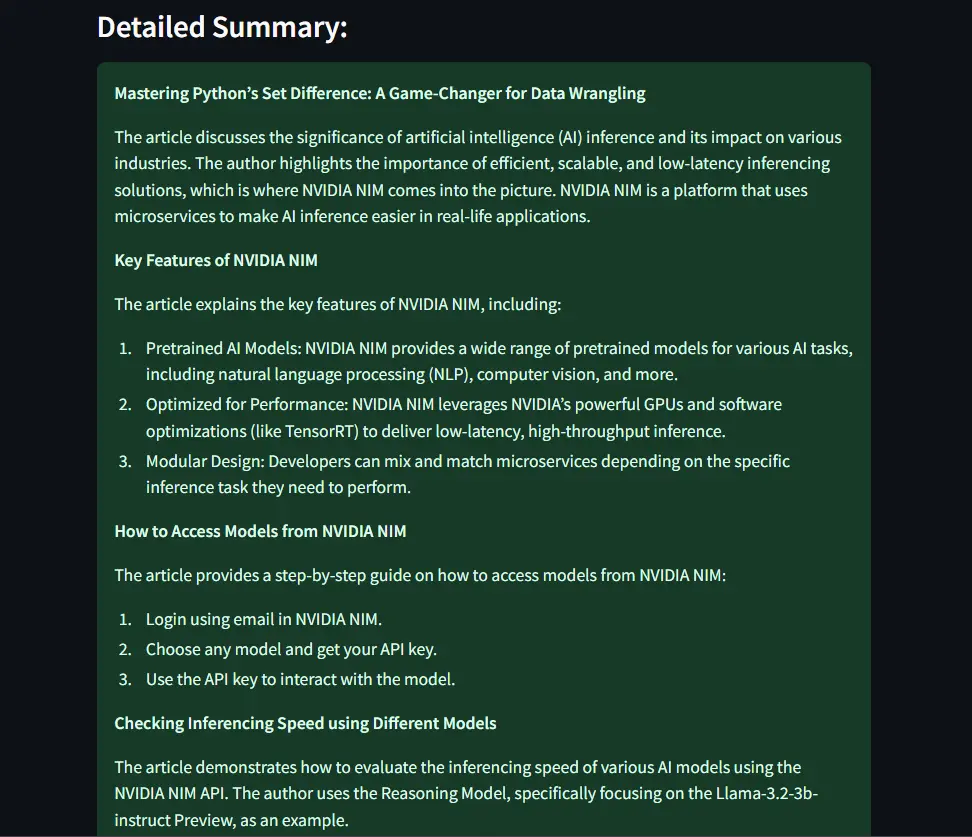
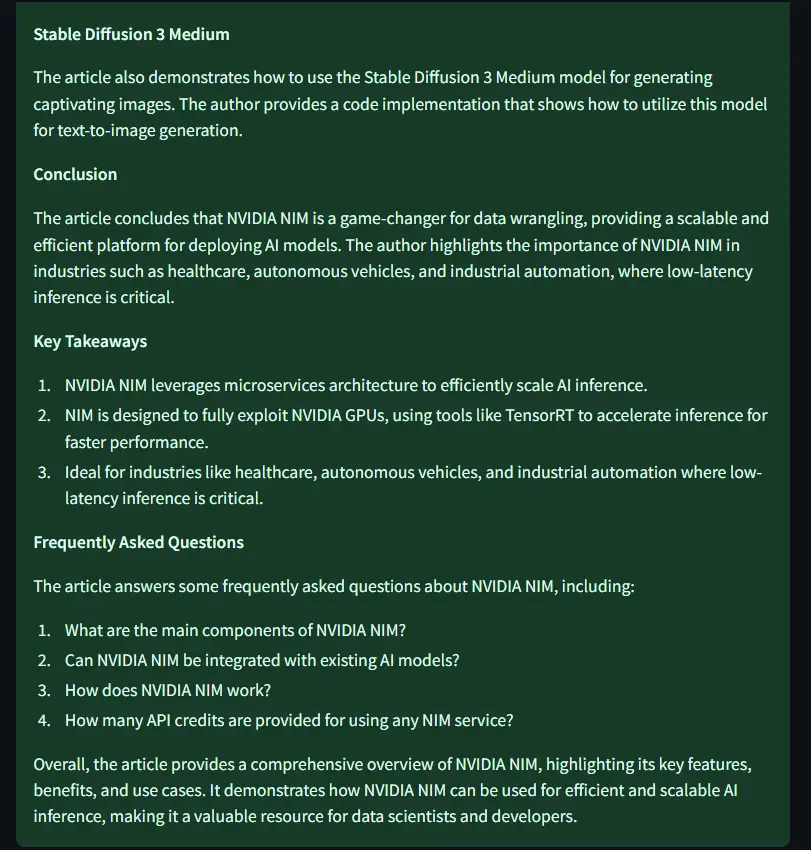
YouTube Video Abstract
Enter video:
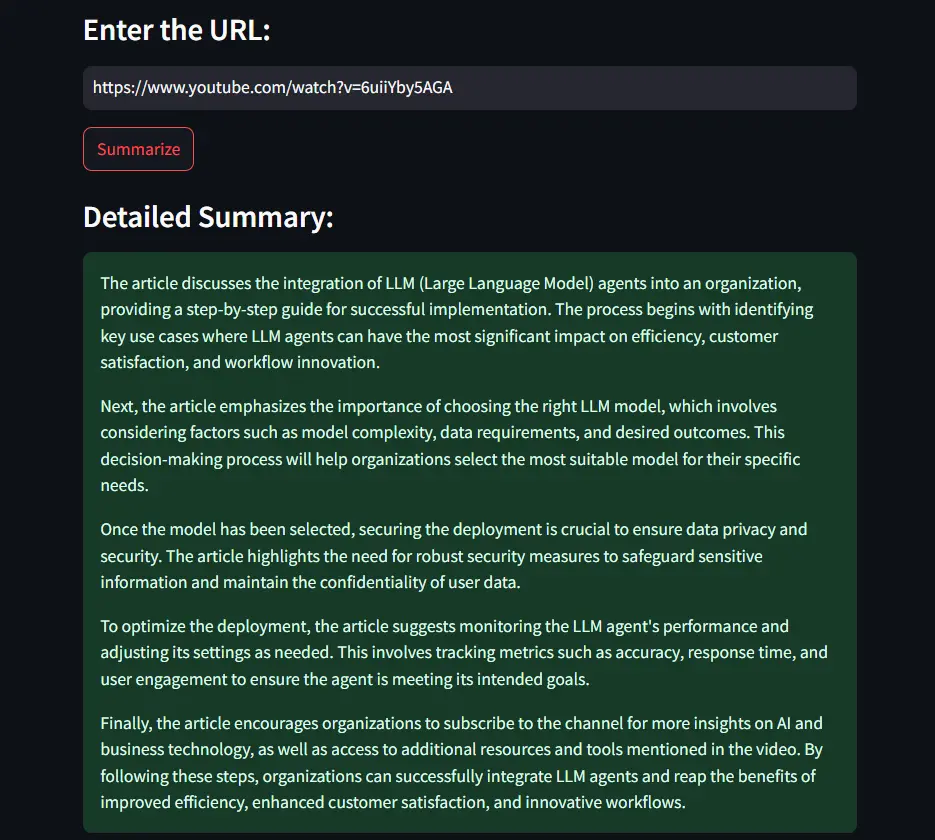
Conclusion
By leveraging the LangChain framework, we streamline interplay with the highly effective Llama 3.2 language mannequin, enabling the era of high-quality summaries. Streamlit made it simple to develop an intuitive and easy-to-use net utility, making the abstract software accessible and interesting.
In conclusion, the article gives a sensible strategy and helpful concepts for making a complete abstract software. By combining cutting-edge language fashions with environment friendly frameworks and easy-to-use interfaces, we are able to open up new potentialities to facilitate data consumption and enhance data acquisition in as we speak’s content-rich world.
Key takeaways
- LangChain facilitates improvement by offering a constant strategy to interacting with language fashions, managing prompts, and chaining processes.
- Groq API’s Llama 3.2 mannequin demonstrates sturdy capabilities in understanding and condensing data, leading to correct and concise summaries.
- The combination of instruments like yt-dlp and UnstructuredURLLoader permits the applying to deal with content material from varied sources corresponding to YouTube and net articles simply.
- The online summarizer makes use of LangChain and Streamlit to offer quick and correct summaries of YouTube movies and web sites.
- Leveraging the Llama 3.2 mannequin, the online summarizer effectively condenses advanced content material into easy-to-understand summaries.
Ceaselessly requested questions
A. LangChain is a framework that simplifies interplay with massive language fashions. It helps handle prompts, chain operations, and entry a number of LLMs, making it simple to create functions like this summarizer.
A. Llama 3.2 generates high-quality textual content and excels at understanding and condensing data, making it properly fitted to abstract duties. It is usually an open supply mannequin.
A. Whereas it could actually deal with a variety of content material, there are limitations. Extraordinarily lengthy movies or articles could require further options corresponding to audio transcription or textual content splitting for optimum summaries.
A. Presently sure. Nonetheless, future enhancements might embody language choice for broader applicability.
A. You should run the supplied code in a Python surroundings with the required libraries put in. See GitHub for the total code and necessities.txt.
The media proven on this article isn’t the property of Analytics Vidhya and is used on the writer’s discretion.